Author |
Message |
Keripo Test Account
Contributor
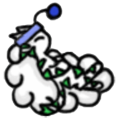
Joined: 11 Apr 2006
Location: Ontario, Canada
|
Posted:
Wed Nov 14, 2007 11:32 pm |
  |
I'm currently creating a modified podzilla 2 build with a few custom functions. The first is loading modules from different locations specified by the passed argments (ie "Podzilla2-Mod /usr/lib/Media /usr/lib/Misc" loads modules from both the "/usr/lib/Media" and "/usr/lib/Misc" folders, and any other ones I may add on). I've already got that implemented and fully working. The second one is menu path sorting based on the module's current folder location. While I have things working nicely at the moment, due to my lack of string manipulation knowledge in C, I'm pretty sure my code at the moment is quite ineffective (memory-wise and probably in other aspects too).
My added code: (note that "MODULEDIR" is defined elsewhere as "/usr/lib"
Code: | // KERIPO MOD
// Module sub-menu based on data path
static char menupath_mod[128]; // Should be suffice space
static char buffer[128];
static char menupath_new[128];
void clear_menupath_mod() {
// Clear everything
strncpy(menupath_mod, "/", strlen(menupath_mod));
strncpy(buffer, "/", strlen(buffer));
}
void set_menupath_mod(PzModule *mod) {
clear_menupath_mod(); // Safeguard
if (strstr(pz_module_get_datapath(mod, ""), MODULEDIR) != NULL) {
// Start from third '/' to second last char, excluding beginning "/usr/lib" part and ending '/'
strncpy(
buffer,
strchr(strchr(strchr(pz_module_get_datapath(mod, ""), '/')+1,'/')+1,'/'),
strlen(strchr(strchr(strchr(pz_module_get_datapath(mod, ""), '/')+1,'/')+1,'/'))-1
);
pz_error("buffer: %s", buffer); // Testing
// Third '/' to second last '/', excluding beginning "/usr/lib" part and ending module name
strncpy(
menupath_mod,
buffer,
strlen(buffer) - strlen(strrchr(buffer, '/'))+1
);
pz_error("menupath_mod: %s", menupath_mod); // Testing
} // Note: if MODULEDIR isn't in the datapath, menupath_mod will still be "/"
}
const char *newpath (const char *menupath) {
// Because sticking everything under "/Extras/" is borrrrring ; )
if (strstr(menupath, "/Extras/") != NULL) {
// Clear things up
strncpy(menupath_new, "/", strlen(menupath_new));
// Add the module's converted menu path
strcpy(menupath_new, menupath_mod);
if (
// Submenus of SVN modules
strstr(menupath, "/Extras/Demos/") != NULL
|| strstr(menupath, "/Extras/Games/") != NULL
|| strstr(menupath, "/Extras/Utilities/") != NULL
) {
// After the "/Extras/Blah/"
strcat(menupath_new, strchr(strchr(strchr(menupath, '/')+1, '/')+1, '/')+1);
} else {
// After the "/Extras/"
strcat(menupath_new, strchr(strchr(menupath, '/')+1, '/')+1);
}
pz_error("menupath_new: %s", menupath_new); // Testing
return menupath_new;
} else {
// Not "/Extras/"
return menupath;
}
} |
In the module.c file, I've replaced "do_init (c->mod);" with:
Code: | set_menupath_mod(c->mod); // So cheap ; P
do_init (c->mod);
clear_menupath_mod(); |
Basically before I load the module, I set the "menupath_mod" variable based on the module's current folder location (found with pz_module_get_datapath). When "resolve_menupath" is called by any pz_add_* functions, the menupath is modified so that "/Extras/" is replaced by the module's folder path.
For example, when I run the binary on my iPod, loading the mandelpod module gives the pz_errors:
Code: | buffer: /Media/mandelpod
menupath_mod: /Media/
menupath_new: /Media/MandelPod
menupath_new: /Media/JuliaPod |
while loading the clocks module gives the pz_errors:
Code: | buffer: /Misc/clocks
menupath_mod: /Misc/
menupath_new: /Misc/Clock/Local Clock
menupath_new: /Misc/Clock/World Clock
menupath_new: /Misc/Clock/World TZ
menupath_new: /Misc/Clock/World DST |
and, as intended, there are the working menu items "/Media/MandelPod", "/Misc/Clock/Local Clock", etc.
While everything works nicely as I intended, I'm sure my coding can be vastly cleaned up. The numerous strchr's I used just to get to the third '/' looks really inefficient and I dislike the idea of creating those 128 static chars (though making them non-static just kills memory and freeses PZ2, so don't have much choice here). I'm not a regular C programmer, so I'm not too familiar with all the functions and how to best use them.
Any suggestions on code improvement?
~Keripo |
_________________ Project ZeroSlackr
http://sourceforge.net/projects/zeroslackr/
http://ipodlinux.org/forums/viewtopic.php?t=29636 |
|
      |
 |
zacaj

Joined: 13 Jul 2006
Location: alone. :(
|
Posted:
Wed Nov 14, 2007 11:46 pm |
  |
So your version puts modules in menus based on thier filesystem location? PLEASE send my the source if so |
_________________ If you have a problem, or want to chat,
PM ME
http://zacaj.com/donate.html |
|
     |
 |
Keripo Test Account
Contributor
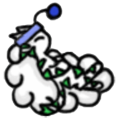
Joined: 11 Apr 2006
Location: Ontario, Canada
|
Posted:
Thu Nov 15, 2007 12:02 am |
  |
zacaj wrote: | So your version puts modules in menus based on thier filesystem location? PLEASE send my the source if so |
Basically yes. I've also got things so that sorting with groups works and all. As for the source, its a series of patch files that'll get uploaded to the ZeroSlackr SVN once I get time. Sending the source files won't be much use as all my changes are grouped together in patch files (a total of 10 patch files I believe, with quite a whole handful of modifications such as these two). Just these two modifications affect four .c files I believe (and thus I use four patch files that also have other modifications for other changes). |
_________________ Project ZeroSlackr
http://sourceforge.net/projects/zeroslackr/
http://ipodlinux.org/forums/viewtopic.php?t=29636 |
|
      |
 |
|
|
View next topic
View previous topic
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
Powered by phpBB
© 2001, 2002 phpBB Group :: FI Theme ::
All times are GMT
|