Author |
Message |
Keripo Test Account
Contributor
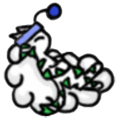
Joined: 11 Apr 2006
Location: Ontario, Canada
|
Posted:
Sat Jan 19, 2008 8:01 pm |
  |
For my Computer Info Sci culminating project I wrote up a small package
manager for "installing" iPL via the loop-mount method. It basically
just installs Loader 2 via ipodpatcher and extracts archives to your
iPod. Due to its rushed nature (i.e. project to be handed in on Monday)
it is far from complete and I won't even bother listing what else needs to be done/added.
For testing purposes, it downloads a packlist from here
and installs a minimal ZeroSlackr system (a very old version with
outdated components but works for demo purposes). At this stage it
doesn't even differentiate between a kernel archive and a pack archive.
All it does is call ipodpatcher and extract files. Really just a bunch
of code slapped together for now.
The package managing part of the program I can fix and improve. The only
real problem I have is iPod detection. Since I only need to demonstrate
that the program roughly works on Windows and doesn't need to be
perfect (for the culminating project at least), my iPod detection code
is just a loop through all the drives looking for the "iPod_Control"
folder. I've looked at both the ipodpatcher and Installer 2 code but my
limited knowledge in both Java-hardware communication and iPod hardware
in general prevents me from really doing much else. Any help in this
area would be greatly appreciated.
So here is the incomplete prototype. The archive is just the Eclipse
project 7-zip'd with compiled jar files in the "export" folder. It is
also just command-line for now (i.e. run it with "java -jar
zeroslackr-lazy-installer-cmd.jar [OPTIONS]" through a command-line) as I
am not too familiar with GUI in Java (though I can always just use one
of those cheap GUI builders like Jigloo). Again this is just a
rushed-but-semi-works version that needs a lot of fixing/cleaning up. It
is not for practical use
yet but just "Software Development" (i.e. looking at the source code
for help/suggestions). Nevertheless, any feedback will be appreciated.
http://www.mediafire.com/download.php?ftcywwiieom
~Keripo |
_________________ Project ZeroSlackr
http://sourceforge.net/projects/zeroslackr/
http://ipodlinux.org/forums/viewtopic.php?t=29636 |
|
      |
 |
Keripo Test Account
Contributor
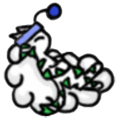
Joined: 11 Apr 2006
Location: Ontario, Canada
|
Posted:
Sat Feb 02, 2008 4:32 am |
  |
New iPod detection code. Unable to test it under a system other than
Windows though (if you can compile for Linux or Mac and can test it,
please do and post the result). In the end I still have to rely on the
"iPod_Control" folder since all Java USB implementations are pretty much
incomplete other than for Linux (i.e. javax.usb and jusb are too much
in their alpha stages).
Here's the Java code
Code: |
/**
* For testing - please reply with your results, thanks!
*/
public static void main(String[] args) {
System.out.println(getHostOS());
System.out.println(getiPodPath());
}
/**
* Convert processed path to native equivalent.
* @param path Path to be converted using '/' as place-holders.
* @return Converted path.
*/
public static String convertPath(String path) {
return path.replace('/', File.separatorChar);
} // convertPath
/**
* Get host OS.
* @return
* -1 if Unsupported <br>
* 1 if Windows <br>
* 2 if Mac OS X <br>
* 3 if Linux <br>
*/
public static int getHostOS() {
String os = System.getProperty("os.name");
if (os.indexOf("Windows") != -1) {
return 1;
} else if (os.indexOf("Mac OS X") != -1) {
return 2;
} else if (os.indexOf("Linux") != -1) {
return 3;
} else {
return -1;
}
} // getHostOS
/**
* Get path to iPod.
* Precondition: iPod is mounted normally
* (i.e. as a removable drive for Windows,
* in "/Volumes/" for Mac OS X,
* or in "/mnt/" for Linux)
* @return Path to iPod's root, null if not found.
*/
public static String getiPodPath() {
String checkDir = "iPod_Control";
File[] roots = null;
switch (getHostOS()) {
case -1: // Unsupported host
break;
case 1: // Windows
roots = File.listRoots();
break;
case 2: // Mac OS X
roots = new File(convertPath("/Volumes/")).listFiles();
break;
case 3: // Linux
roots = new File(convertPath("/mnt/")).listFiles();
break;
}
if (roots != null) {
for (File f: roots) {
String path = f.getAbsolutePath();
if (new File(path + checkDir).exists()
|| new File(path +
File.pathSeparator + checkDir).exists() ) { // FIXME - does this work
for Mac and Linux???
return path;
}
}
}
return null;
} |
|
_________________ Project ZeroSlackr
http://sourceforge.net/projects/zeroslackr/
http://ipodlinux.org/forums/viewtopic.php?t=29636 |
|
      |
 |
Rufus
Moderator

Joined: 28 Apr 2005
Location: Australia
|
Posted:
Thu Feb 07, 2008 1:09 pm |
  |
I made a few changes, and it now works correctly on Linux.
Code: |
import java.io.BufferedReader;
import java.io.File;
import java.io.FileReader;
import java.io.IOException;
import java.util.ArrayList;
/**
*
* @author rufus
*/
public class Main {
/**
* For testing - please reply with your results, thanks!
*/
public static void main(String[] args) {
System.out.println(getHostOS());
System.out.println(getiPodPath());
}
/**
* Convert processed path to native equivalent.
* @param path Path to be converted using '/' as place-holders.
* @return Converted path.
*/
public static String convertPath(String path) {
return path.replace('/', File.separatorChar);
} // convertPath
/**
* Get host OS.
* @return
* -1 if Unsupported <br>
* 1 if Windows <br>
* 2 if Mac OS X <br>
* 3 if Linux <br>
*/
public static int getHostOS() {
String os = System.getProperty("os.name");
if (os.indexOf("Windows") != -1) {
return 1;
} else if (os.indexOf("Mac OS X") != -1) {
return 2;
} else if (os.indexOf("Linux") != -1) {
return 3;
} else {
return -1;
}
} // getHostOS
/**
* Get path to iPod.
* Precondition: iPod is mounted normally
* (i.e. as a removable drive for Windows,
* in "/Volumes/" for Mac OS X,
* or in "/mnt/" for Linux)
* @return Path to iPod's root, null if not found.
*/
public static String getiPodPath() {
String checkDir = "iPod_Control";
File[] roots = null;
switch (getHostOS()) {
case -1: // Unsupported host
break;
case 1: // Windows
roots = File.listRoots();
break;
case 2: // Mac OS X
roots = new File(convertPath("/Volumes/")).listFiles();
break;
case 3: // Linux
try {
BufferedReader reader = new BufferedReader(new
FileReader("/proc/mounts"));
ArrayList<String> list = new ArrayList();
for(;;) {
String line = reader.readLine();
if(line == null) break;
int ispace = line.indexOf(' ');
list.add(line.substring(ispace+1, line.indexOf(' ',
ispace+1)));
}
reader.close();
roots = new File[list.size()];
for(int i=0; i<list.size(); i++)
roots[i] = new File(list.get(i));
} catch (IOException ex) {
File[] mnt = new File("/mnt/").listFiles();
File[] media = new File("/media/").listFiles();
roots = new File[mnt.length + media.length];
System.arraycopy(mnt, 0, roots, 0, mnt.length);
System.arraycopy(media, 0, roots, mnt.length, media.length);
}
}
if (roots != null) {
for (File f: roots) {
String path = f.getAbsolutePath();
if (new File(path + checkDir).exists()
|| new File(path
+ File.separator + checkDir).exists() ) { // FIXME - does this work for
Mac and Linux???
return path;
}
}
}
return null;
}
} |
Edit:
Oh, and the output is:
3
/media/IPOD
Edit 2:
Whoops, fixed some obviously broken fall-back code if the procfs is not mounted at /proc or is unavailable. |
_________________ Do not PM me with questions about installing things. |
|
   |
 |
Keripo Test Account
Contributor
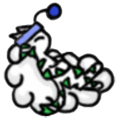
Joined: 11 Apr 2006
Location: Ontario, Canada
|
Posted:
Sat Feb 09, 2008 2:40 am |
  |
Thanks mucho Rufus. I played around with your code and re-did using
/proc/mounts (never knew it existed before). I prefer Scanner over
BufferedReader as thats what I'm more use to. I also didn't realize I
used pathSeparatorChar instead of separatorChar (whoops). I also had to
change how checking/returning is done since Windows returns it with the
unwanted extra "\" (all my extract methods start with a separatorChar).
Code: |
/**
* Get path to iPod.
* Precondition: iPod is mounted
* (i.e. as a removable drive for Windows,
* in "/Volumes/" for Mac OS X,
* or in somewhere for Linux)
* @return Path to iPod's root, null if not found.
*/
public static String getiPodPath() {
final String CHECK_DIR = "iPod_Control";
File[] roots;
switch (getHostOS()) {
case -1: // Unsupported host
break;
case 1: // Windows
roots = File.listRoots();
for (File f: roots) {
String path = f.getAbsolutePath();
if (new File(path + CHECK_DIR).exists()) {
// Need to remove the ending "\"
return path.substring(0, path.length()-1);
}
}
break;
case 2: // Mac OS X
roots = new File(Tools.convertPath("/Volumes/")).listFiles();
for (File f: roots) {
String path = f.getAbsolutePath();
if (new File(path + File.separatorChar + CHECK_DIR).exists()) {
return path;
}
}
break;
case 3: // Linux - Thanks mucho to Rufus!
try {
Scanner proc = new Scanner(new File("/proc/mounts"));
String procLine;
ArrayList<File> mounts = new ArrayList<File>();
while (proc.hasNextLine()) {
procLine = proc.nextLine();
int i = procLine.indexOf(' ') + 1;
mounts.add(new File(procLine.substring(i, procLine.indexOf(' ',
i))));
}
proc.close();
roots = new File[mounts.size()];
roots = mounts.toArray(roots);
} catch (IOException e) {
File[] mnt = new File("/mnt/").listFiles();
File[] media = new File("/media/").listFiles();
roots = new File[mnt.length + media.length];
System.arraycopy(mnt, 0, roots, 0, mnt.length);
System.arraycopy(media, 0, roots, mnt.length, media.length);
}
for (File f: roots) {
String path = f.getAbsolutePath();
if (new File(path + File.separatorChar + CHECK_DIR).exists()) {
return path;
}
}
break;
} //switch
return null;
} // getiPodPath |
Tested it on Slax and it worked fine (returned "/mnt/sda2_removable"). I
can't test it on Mac but, assuming that the iPod always gets mounted to
/Volumes/, it should work fine. |
_________________ Project ZeroSlackr
http://sourceforge.net/projects/zeroslackr/
http://ipodlinux.org/forums/viewtopic.php?t=29636 |
|
      |
 |
Rufus
Moderator

Joined: 28 Apr 2005
Location: Australia
|
Posted:
Sat Feb 09, 2008 4:52 pm |
  |
No worries, also, on Linux, and presumably other UNIXes, redundant
separators are ignored, so a path like //media////disk/// is equivalent
to /media/disk/, not sure about Windows though.
Oh, and OSX may well have an equivalent to /proc, I'll have a look next time I'm near a Mac. |
_________________ Do not PM me with questions about installing things. |
|
   |
 |
|
|
View next topic
View previous topic
You cannot post new topics in this forum You cannot reply to topics in this forum You cannot edit your posts in this forum You cannot delete your posts in this forum You cannot vote in polls in this forum
|
Powered by phpBB
© 2001, 2002 phpBB Group :: FI Theme ::
All times are GMT
|